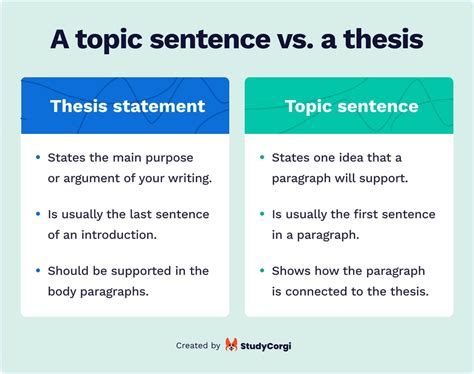
The highly anticipated Summer Finance Internship 2025 is now open for applications, offering an exceptional opportunity for aspiring finance professionals to gain invaluable firsthand experience in the dynamic world of finance. This prestigious program, designed for exceptional undergraduate students, provides a comprehensive immersion into the core principles and practices of the industry, under the guidance of experienced mentors and industry experts. With a focus on developing both technical skills and professional acumen, the internship promises to accelerate your finance career and provide you with a competitive edge in the job market.
Throughout the summer-long program, interns will embark on a tailored learning journey, participating in a wide range of projects and assignments that reflect real-world business scenarios. From financial modeling and analysis to investment research and portfolio management, you will gain a deep understanding of the various facets of finance, honing your analytical, problem-solving, and decision-making abilities. Moreover, you will have the opportunity to engage with industry leaders, attend exclusive workshops and seminars, and network with professionals from leading financial institutions, providing you with invaluable insights and connections.
Beyond technical knowledge and skills, the Summer Finance Internship 2025 is committed to fostering your professional development. You will receive dedicated mentorship from experienced finance professionals who will guide you through the program and provide personalized feedback. Furthermore, you will participate in workshops on leadership, communication, and professional ethics, developing the soft skills that are essential for success in the finance industry. By the end of the program, you will have not only acquired a solid foundation in finance but also honed the professional qualities that will enable you to excel in your career.
Navigating the 2025 Summer Finance Internship Landscape
Early Preparation: A Key to Success
To maximize your chances of securing a coveted summer finance internship in 2025, early preparation is paramount. Begin researching target companies and industries, delving into their values, operations, and internship programs. Identify key individuals within these organizations, such as hiring managers and alumni, and build connections through networking events, online platforms, and informational interviews.
Furthermore, hone your technical skills through coursework, online certifications, and industry-specific workshops. Enhance your financial modeling, data analysis, and presentation abilities. Seek opportunities to develop your teamwork, communication, and analytical thinking skills through extracurricular activities, such as finance clubs or consulting projects.
Building a Competitive Profile
Craft a strong resume that showcases your relevant skills, experiences, and academic achievements. Highlight your passion for finance, analytical prowess, and commitment to the industry. Tailor your resume and cover letter to each internship application, emphasizing specific aspects that align with the company’s requirements.
Prepare thoroughly for technical interviews by practicing valuation, financial modeling, and behavioral questions. Engage in mock interviews with peers, mentors, or career counselors to refine your communication and problem-solving abilities. Additionally, demonstrate your leadership, initiative, and interest in the financial sector through extracurricular activities, research projects, and volunteer work.
Keys to Unlocking Top Finance Internship Opportunities
Research and Identify Target Companies
Thoroughly research the financial industry to identify potential employers that align with your interests and career goals. Attend industry events, connect with professionals on LinkedIn, and consult financial publications to gain insights into different companies and their internship programs.
Build a Strong Profile
Develop a compelling resume and cover letter that showcase your academic achievements, relevant skills, and passion for finance. Participate in extracurricular activities, leadership roles, or research projects to demonstrate your commitment to the field and your ability to perform in a demanding environment.
Network Effectively
Attend industry networking events, reach out to hiring managers directly, and connect with alumni or industry professionals on LinkedIn. Build relationships with individuals who can provide insights into the internship application process, offer guidance, and potentially refer you for open positions.
Preparation for Technical and Behavioral Interviews
Technical Interviews
Review core finance concepts, including financial modeling, valuation, and accounting principles. Practice solving case studies and numerical problems to demonstrate your analytical skills and problem-solving abilities.
Behavioral Interviews
Prepare for common behavioral interview questions by reflecting on your experiences, skills, and motivations. Emphasize your work ethic, teamwork capabilities, and drive to succeed in a competitive environment.
Additional Tips
* Apply early to increase your chances of being considered.
* Tailor your application to each specific company and role.
* Proofread your application materials carefully for any errors.
* Follow up with the hiring manager to express your interest and reiterate your qualifications.
Emerging Trends in Summer Finance Internships
Summer finance internships are a valuable opportunity for students to gain hands-on experience in the field and make connections that can lead to future employment. In recent years, several emerging trends have shaped the nature of these internships:
Increased Focus on Technology
Advancements in technology, such as artificial intelligence and blockchain, are revolutionizing the finance industry. Internships that provide exposure to these technologies are becoming increasingly sought-after, as employers seek individuals with the skills and knowledge to navigate the rapidly evolving landscape.
Emphasis on Sustainability and ESG
There is a growing awareness of the impact of financial decisions on environmental, social, and governance (ESG) factors. Internships that focus on sustainability and ESG provide students with the opportunity to learn about responsible investing and corporate social responsibility.
Virtual and Hybrid Formats
The COVID-19 pandemic has accelerated the trend towards virtual and hybrid internships. These formats offer greater flexibility for students and employers, allowing remote participation and a mix of in-person and online experiences.
Trend |
Examples |
Increased Focus on Technology |
Internships in AI development, FinTech, and data analysis |
Emphasis on Sustainability and ESG |
Internships in impact investing, clean energy, and social finance |
Virtual and Hybrid Formats |
Remote internships, rotational programs with both in-office and virtual components |
The Role of Artificial Intelligence in Internship Hiring
Artificial intelligence (AI) is rapidly transforming the world of recruitment, and internship hiring is no exception. AI-powered tools are being used to automate various tasks throughout the internship hiring process, from screening resumes and cover letters to scheduling interviews and making hiring decisions.
Improved Efficiency
AI algorithms can quickly and efficiently scan through large volumes of applications, identifying candidates who meet the minimum qualifications for the internship. This frees up hiring managers from having to manually review each application, saving them time and effort.
Unbiased Selection
AI removes human bias from the internship hiring process. AI algorithms are not influenced by factors such as a candidate’s gender, race, or age. This helps ensure that all candidates are evaluated fairly and based solely on their qualifications.
Candidate Engagement
AI-powered chatbots can engage with candidates throughout the internship hiring process. These chatbots can answer questions, provide guidance, and schedule interviews. This helps create a positive candidate experience and makes the internship hiring process more transparent.
Additional Benefits of AI in Internship Hiring
In addition to the above benefits, AI can also help streamline the internship hiring process, reduce costs, and improve decision-making. Here is a table summarizing the additional benefits of AI in internship hiring:
Benefit |
Description |
Streamlined Process |
AI automates tasks, making the internship hiring process more efficient and faster. |
Reduced Costs |
AI can reduce the cost of internship hiring by automating tasks and reducing the need for human recruiters. |
Improved Decision-Making |
AI algorithms can provide hiring managers with data-driven insights to help them make better hiring decisions. |
Strategies for a Standout Summer Finance Internship Application
As you prepare your summer finance internship application in 2025, consider these strategies to enhance your chances of success:
Research and Target Specific Firms
Identify companies and industries that align with your interests and career aspirations. Thoroughly research their culture, values, and internship programs.
Craft a Compelling Resume and Cover Letter
Highlight your relevant skills and experiences, quantifying accomplishments whenever possible. Tailor your cover letter to each firm, expressing your enthusiasm and why you’re a suitable candidate.
Network and Get Referrals
Attend industry events and connect with professionals in your desired field. Ask for introductions and recommendations that can strengthen your application.
Prepare for Technical Interviews
Practice solving financial modeling problems, evaluating investment opportunities, and analyzing market trends. Showcase your analytical, problem-solving, and communication abilities.
Demonstrate Your Passion and Fit
Convey your genuine interest in the finance industry and how the firm’s values resonate with you. Share examples of your extracurricular activities, research projects, or volunteer experiences that demonstrate your commitment and teamwork skills.
Skill |
Example |
Financial Modeling |
Built financial models to analyze investment opportunities and prepare presentations for senior executives |
Market Analysis |
Monitored market trends, identified investment opportunities, and presented recommendations to portfolio managers |
Deal Execution |
Assisted in executing M&A transactions, including due diligence, valuation, and negotiation |
By implementing these strategies, you can increase your chances of securing a top-notch summer finance internship that will enhance your professional development and career trajectory.
Professional Development Opportunities in Summer Finance Internships
Technical Skills Enhancement
Interns gain hands-on experience in various finance functions, such as financial analysis, modeling, and investment management. They develop analytical, quantitative, and problem-solving skills that are essential in the finance industry.
Industry Knowledge Acquisition
Internships provide exposure to different areas of finance and the workings of financial institutions. Interns learn about market trends, financial regulations, and best practices in the industry.
Networking Opportunities
Internships offer a platform for interns to interact with industry professionals, including mentors, colleagues, and clients. They build valuable connections that can enhance their career prospects.
Mentorship and Guidance
Interns typically receive mentorship and guidance from experienced finance professionals. They learn from the expertise of their mentors, gain valuable insights, and develop their interpersonal and communication skills.
Soft Skill Development
Internships foster the development of soft skills such as teamwork, communication, and time management. Interns learn to collaborate effectively, present their ideas clearly, and meet deadlines under pressure.
Career Exploration and Advancement
Internships provide interns with an opportunity to explore different career paths in finance. They gain a better understanding of their interests and capabilities, and they can make informed decisions about their future careers.
Skill |
Development Opportunities |
Financial analysis |
Conduct financial modeling, perform due diligence, and analyze investment opportunities. |
Investment management |
Participate in portfolio management, research investments, and make investment recommendations. |
Valuation and modeling |
Learn various valuation techniques, build financial models, and forecast financial performance. |
The Future of Finance Internships in the Tech-Driven Economy
Evolving Skillsets: From Excel to Python
Finance internships are undergoing a technological transformation, demanding proficiency in programming languages like Python and SQL. These skills enhance data analysis, modeling, and automation capabilities, preparing interns for the tech-driven finance landscape.
Artificial Intelligence and Machine Learning
AI and ML are revolutionizing finance, and internships now incorporate these technologies. Interns gain hands-on experience developing AI-powered trading algorithms, risk assessment models, and fraud detection systems.
Blockchain and Cryptocurrency
The rise of blockchain and cryptocurrencies has created opportunities for internships in these emerging fields. Interns explore the complexities of distributed ledger technology, crypto asset management, and blockchain-based financial applications.
Data Analytics and Visualization
Financial data is now analyzed with sophisticated tools and visualization techniques. Internships expose interns to data mining, statistical modeling, and interactive visualizations, enabling them to draw insights from complex datasets.
Robo-Advisors and Financial Automation
Robo-advisors and other automated financial tools are transforming wealth management. Internships provide experience in designing, testing, and deploying these technologies, fostering a deep understanding of automated financial services.
FinTech Integration and Collaboration
FinTech startups are collaborating with traditional financial institutions. Internships in this area offer a blend of exposure to financial markets and cutting-edge technology, preparing interns for the evolving financial ecosystem.
Table: Skills Required for Future Finance Internships
Technical |
Soft |
Python, SQL |
Analytical |
AI, ML |
Problem-Solving |
Blockchain |
Communication |
Data Analytics |
Teamwork |
Robo-Advisors |
Business Acumen |
FinTech |
Networking |
Financial Modeling and Data Analytics in Summer Finance Internships
Financial Modeling
Financial modeling plays a pivotal role in summer finance internships, providing insights into complex financial scenarios. Interns gain hands-on experience using specialized software such as Excel and proprietary systems to create financial models that assess the financial performance and make investment decisions.
Data Analytics
Data analytics is another key aspect of summer finance internships. Interns learn to analyze large financial datasets using statistical techniques and programming languages like Python and R. They identify trends, patterns, and anomalies that help decision-making and risk management.
Mergers and Acquisitions (M&A)
M&A internships involve analyzing potential acquisition targets, conducting due diligence, and preparing financial models to evaluate the viability of deals. Interns gain a deep understanding of deal structuring, valuation techniques, and negotiation strategies.
Sales and Trading
Sales and trading internships offer exposure to the fast-paced world of financial markets. Interns learn about different asset classes, trading strategies, and risk management. They also develop communication and interpersonal skills through interactions with clients and market participants.
Asset Management
Asset management internships focus on managing portfolios of stocks, bonds, or other assets. Interns assist with investment research, portfolio construction, and performance monitoring. They gain experience in portfolio diversification, risk assessment, and client communication.
Investment Banking
Investment banking internships provide comprehensive training in financial advisory roles. Interns work on mergers and acquisitions, capital raising, and debt restructuring transactions. They learn about financial structuring, valuation, and negotiation.
Private Equity
Private equity internships involve investing in private companies with the goal of growth and eventual exit through an initial public offering or sale to a strategic acquirer. Interns contribute to due diligence, portfolio management, and exit strategies.
FinTech
FinTech internships focus on the intersection of technology and finance. Interns work on developing innovative financial products and services, leveraging artificial intelligence, blockchain, and data analytics. They gain exposure to the latest trends in financial technology and its impact on the industry.
Intercultural Experiences in International Finance Internships
International finance internships offer unique opportunities for cultural immersion and personal growth. Here are some specific examples of potential intercultural experiences:
Language Skills Enhancement
Interning in a country with a different native language can significantly improve your language proficiency through daily interactions and immersion in the local culture.
Cultural Etiquette and Communication Styles
Navigating business meetings, social situations, and everyday life requires understanding and adapting to different cultural communication styles and etiquette norms.
Professional Networking
Interning abroad allows you to build relationships with professionals from diverse backgrounds, expanding your global network and broadening your career horizons.
Global Market Awareness
Gaining firsthand experience in international financial markets provides insights into global economic trends, financial regulations, and investment strategies.
Adaptability and Resilience
Adapting to a new culture, navigating cultural differences, and solving problems in unfamiliar environments fosters adaptability and resilience.
Cultural Exchange and Understanding
Intercultural experiences promote mutual understanding and appreciation between people from different cultures, breaking down stereotypes and promoting tolerance.
Historical and Cultural Immersion
Many international financial centers are home to rich historical and cultural heritage, offering opportunities to explore and learn about different civilizations and ways of life.
Personal Growth and Maturity
Stepping outside your comfort zone and immersing yourself in a new culture challenges your preconceptions, fosters self-reflection, and promotes personal growth.
Career Advantages
Intercultural experiences enhance your resume, demonstrate your adaptability, and set you apart as a highly desirable candidate in the global finance industry.
Experience |
Benefits |
Language Skills |
Enhancement of language proficiency |
Etiquette |
Understanding of cultural norms |
Networking |
Expansion of global connections |
Global Market |
Insights into global trends |
Adaptability |
Fostering of resilience |
Cultural Exchange |
Promotion of understanding |
Historical Immersion |
Exploration of diverse cultures |
Personal Growth |
Challenge of preconceptions |
Career Advantages |
Enhancement of resume |
Blockchain and Cryptocurrency in Summer Finance Internships
The rapid growth and transformative potential of blockchain and cryptocurrency have made them increasingly relevant in the financial industry. Summer finance internships offer aspiring professionals an opportunity to gain hands-on experience in this cutting-edge field.
Exploring Blockchain Technology
Interns may have the opportunity to work on projects involving:
- Developing and implementing blockchain solutions for supply chain management or trading platforms.
- Examining the regulatory and compliance aspects of blockchain applications.
- Analyzing and evaluating the potential impact of blockchain on financial markets.
Immersion in Cryptocurrency Markets
Internships can provide participants with insights into:
- Trading and investing strategies for digital assets.
- Building and maintaining cryptocurrency exchanges.
- Exploring the role of stablecoins and decentralized finance in the financial system.
Table: Summer Finance Internships with Blockchain and Cryptocurrency Focus
| Institution | Location | Focus Areas |
|—|—|—|
| Goldman Sachs | New York | Blockchain development, digital asset trading |
| BlackRock | San Francisco | Cryptocurrency investment management, blockchain solution design |
| Coinbase | Remote | Cryptocurrency exchange operations, blockchain research |
Emerging Technologies and Innovations
Summer internships allow interns to engage with:
- The latest advances in blockchain technology, such as smart contracts and distributed ledger technology.
- Innovative applications of cryptocurrency in fields like decentralized finance, data security, and gaming.
- Emerging trends in regulatory frameworks for blockchain and cryptocurrency.
Synopsis: Summer Finance Internship 2025
The Summer Finance Internship 2025 is a competitive program designed to provide highly motivated students with valuable hands-on experience in the financial industry. The internship will offer a comprehensive overview of various aspects of finance, including investment banking, asset management, and financial analysis. Interns will gain practical knowledge, develop their analytical skills, and build a network of professionals in the field.
The program is open to undergraduate and graduate students pursuing degrees in finance, economics, business, and related fields. Applicants must demonstrate a strong academic record, a keen interest in finance, and exceptional communication and interpersonal skills. The internship will take place at a leading financial institution in a major financial center and will include a mix of project work, presentations, and networking opportunities.
Upon completion of the internship, participants will be well-prepared to pursue careers in the financial industry. They will have gained a deep understanding of financial principles, developed their analytical and problem-solving abilities, and expanded their professional network. The Summer Finance Internship 2025 is an invaluable opportunity for students to launch their careers in finance.
People Also Ask
What are the eligibility requirements for the Summer Finance Internship 2025?
To be eligible for the Summer Finance Internship 2025, applicants must:
- Be an undergraduate or graduate student pursuing a degree in finance, economics, business, or a related field.
- Have a strong academic record with a GPA of 3.5 or higher.
- Demonstrate a keen interest in finance through coursework, research, or extracurricular activities.
- Possess exceptional communication and interpersonal skills.
What is the application process for the Summer Finance Internship 2025?
The application process for the Summer Finance Internship 2025 typically includes the following steps:
- Submit an online application, including your resume, transcript, and a cover letter.
- Complete online assessments, such as a logical reasoning test and a situational judgment test.
- Participate in one or more rounds of virtual or in-person interviews with the hiring team.
What are the benefits of participating in the Summer Finance Internship 2025?
The benefits of participating in the Summer Finance Internship 2025 include:
- Gaining valuable hands-on experience in the financial industry.
- Developing analytical and problem-solving skills.
- Building a network of professionals in the field.
- Learning about different career paths in finance.
- Preparing for a successful career in the financial industry.